Ever wanted to discover new books in a fun and creative way? That’s exactly why I created the Twitter Bot Book project—a bot that tweets daily book recommendations based on the word of the day. It was an exciting experiment combining APIs, automation, and some lighthearted creativity.
📌 The Idea
The goal was simple: use automation to help people find books in a quirky, unexpected way. Every day, the bot:
- Generates a random word (e.g., "adventure").
- Fetches book data related to the word from the Open Library API.
- Posts a tweet with the book's details, including its cover image.
It’s a fun, daily dose of literary inspiration—perfect for bookworms or anyone looking to read more.
🛠️ Tech Stack
Here’s what powered the project:
- Node.js: The backbone of the bot, for writing JavaScript.
- Twitter API: To automate tweeting.
- Open Library API: For fetching book data.
- RapidAPI (Random Word API): For generating the word of the day.
🚀 How It Works
Step 1: Setup and Configuration
The bot uses environment variables to store API credentials securely. Here’s an example .env
file:
TWITTER_CONSUMER_KEY=your_consumer_key
TWITTER_CONSUMER_SECRET=your_consumer_secret
TWITTER_ACCESS_TOKEN=your_access_token
TWITTER_ACCESS_TOKEN_SECRET=your_access_token_secret
RAPID_API_WORDS=your_rapid_api_token
Step 2: Fetching the Word of the Day
The bot generates a random word using the RapidAPI service:
const axios = require("axios");
const getRandomWord = async () => {
const response = await axios.get("https://random-word-api.p.rapidapi.com", {
headers: { "X-RapidAPI-Key": process.env.RAPID_API_WORDS },
});
return response.data.word;
};
Step 3: Finding a Book
It then searches the Open Library API for books matching the word:
const searchBook = async (word) => {
const response = await axios.get(`https://openlibrary.org/search.json?q=${word}`);
return response.data.docs[0]; // Returns the first match
};
Here is an example for the word of the day "Beeswax":
{
"author_key": [
"OL13202440A",
"OL13202441A"
],
"author_name": [
"Tessa Jensen",
"Liz D. Hill"
],
"ebook_access": "no_ebook",
"ebook_count_i": 0,
"edition_count": 2,
"edition_key": [
"OL49601854M",
"OL49601859M"
],
"first_publish_year": 2023,
"has_fulltext": false,
"isbn": [
"9798985832655",
"9798985832648"
],
"key": "/works/OL36727042W",
"language": [
"eng"
],
"last_modified_i": 1694979176,
"public_scan_b": false,
"publish_date": [
"2023"
],
"publish_year": [
2023
],
"publisher": [
"Jensen, Tessa"
],
"seed": [
"/books/OL49601854M",
"/books/OL49601859M",
"/works/OL36727042W",
"/authors/OL13202440A",
"/authors/OL13202441A",
"/subjects/fiction_general",
"/subjects/fiction_mystery_&_detective_general"
],
"title": "Astrid Beeswax",
"title_suggest": "Astrid Beeswax",
"title_sort": "Astrid Beeswax",
"type": "work",
"subject": [
"Fiction, general",
"Fiction, mystery & detective, general"
],
"publisher_facet": [
"Jensen, Tessa"
],
"subject_facet": [
"Fiction, general",
"Fiction, mystery & detective, general"
],
"_version_": 1795913894679543810,
"author_facet": [
"OL13202440A Tessa Jensen",
"OL13202441A Liz D. Hill"
],
"subject_key": [
"fiction_general",
"fiction_mystery__detective_general"
]
}
Step 4: Tweeting the Recommendation
Finally, the bot formats the book details and posts a tweet using the twit
library:
const Twit = require("twit");
const T = new Twit({ ...your_twitter_keys });
const tweetBook = (book) => {
const tweet = `${emoji.get('book')} ${bookTitle}\n${bookPublishedDate ? `${emoji.get('date')} ${bookPublishedDate}\n` : ''}${bookPages ? `${emoji.get('page_facing_up')} ${bookPages} pages\n` : ''}${bookDescription ? `${emoji.get('memo')} ${bookDescription}\n` : ''}${authorString ? `${authorString}` : ''}\n\n#WordOfTheDay #${wordOfTheDay} #BookOfTheDay #reading`;
T.post("statuses/update", { status: tweet });
};
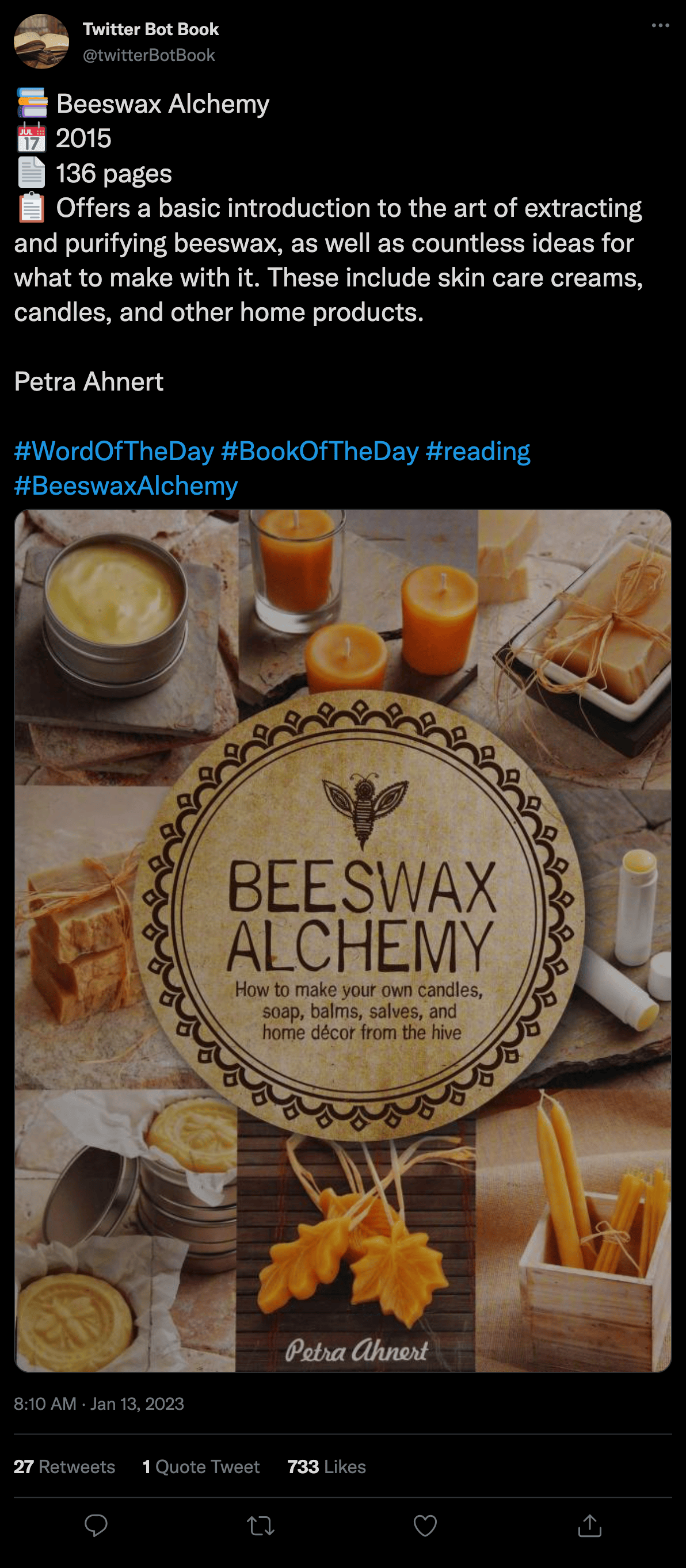
🤔 Challenges and Solutions
Issue: API Rate Limits
Solution: Implemented caching to reduce duplicate API calls.
Issue: Finding Relevant Books
Solution: Added fallback logic for less common words to fetch alternative results.
đź§ Lessons Learned
- APIs Simplify Automation: Open Library and RapidAPI made this project possible.
- Handle Failures Gracefully: Not all words yield relevant books—fallbacks are key.
- Iterate Quickly: Small, daily updates kept the project fun and manageable.
What’s Next?
- Enhance Book Filtering: Improve logic for better matches.
- Improve Visual Content: Generate tweet previews with styled graphics.
- Expand API Options: Include more data sources for diverse recommendations.
Check out the GitHub repository here: twitter-bot-book